Exploring the different data types in Python
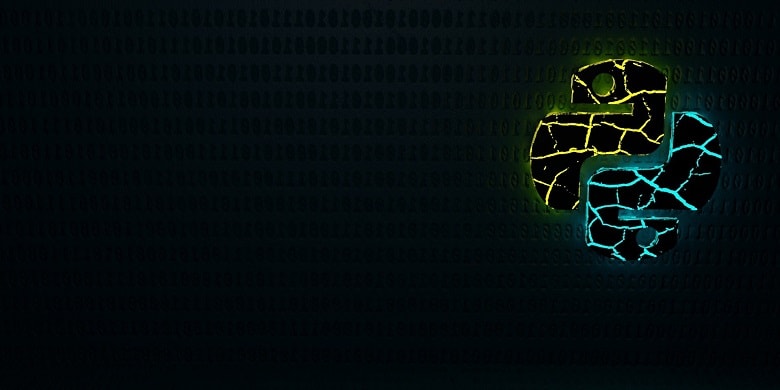
Table of Contents
Introduction
Python is a widely-used, high-level programming language known for its simplicity and flexibility. One of the key features of Python is its support for a variety of data types. One of the benefits of Python is that it can be run on a variety of platforms and compilers including Windows compiler, Mac compiler, online python compiler, and Linux compiler and it is available for free. This means that you don’t need to spend money on expensive software or worry about compatibility issues (this Python VS JavaScript guide will give you more insights).
Table of Contents
In this article, we will explore the different data types available in Python and how they are used to store and manipulate data. From basic data types such as integers and strings to more advanced data types such as lists and dictionaries, we will cover everything you need to know to effectively use data in your Python programs.

Different data types in Python
Numeric types
In Python, there are several different numeric data types that can be used to represent and manipulate numbers. These include:
- int (integer): This is the most basic numeric data type in Python, used to represent whole numbers. Integers can be positive or negative and have no decimal point. Example: 1, -5, 100
- float (floating-point): This data type is used to represent numbers with decimal points. They are often used to represent real numbers. Example: 3.14, -0.5, 1.0
- complex: This data type is used to represent complex numbers, which consist of a real part and an imaginary part. The imaginary part is represented by the letter “j” or “J”. Example: 3 + 4j, -5j, 2 + 0j
- Decimal: This data type is used to represent decimal numbers and it is useful in financial applications, where precision is critical. Decimal numbers are specified using the decimal module in Python. Example: decimal.Decimal(1.5), decimal.Decimal(2)
- Fraction: This data type is used to represent fractions and it is useful in mathematical calculations that involve fractions. Fractions are specified using the fractions module in Python

Sequences
In Python, there are several different sequence data types that can be used to store and manipulate ordered collections of items. These include:
- list: Lists are the most basic and versatile sequence data type in Python. They are used to store an ordered collection of items, which can be of any type. Lists are defined using square brackets [] and items are separated by commas. Example: [1, 2, 3], [‘a’, ‘b’, ‘c’], [1, ‘a’, 3.14]
- tuple: Tuples are similar to lists, but they are immutable, meaning that their items cannot be modified once they are created. Tuples are defined using parentheses () and items are separated by commas. Example: (1, 2, 3), (‘a’, ‘b’, ‘c’), (1, ‘a’, 3.14)
- range: A range is a sequence of numbers that are created using the range() function. Ranges are typically used in loops and other operations that require a sequence of numbers. They are defined by providing a start, stop, and step value. Example: range(5), range(1,5), range(1,5,2)
- str: String is a sequence of characters, it is immutable. You can use single or double quotes to define a string. Example: “Hello”, “world!”, ‘python’
- bytearray: A bytearray is a mutable sequence of integers that represent bytes. They are similar to lists, but they are specifically designed to work with binary data. Example: bytearray(b’Hello’), bytearray(b’world!’)
Sets
In Python, the set data type is used to store an unordered collection of unique items. Sets are defined using curly braces {} or the built-in set() function.
Here are some key features of sets in Python:
- Uniqueness: Sets only store unique items, meaning that if an item is added to a set more than once, it will only be stored once. This makes sets useful for tasks such as removing duplicates from a list.
- Unordered: Sets do not have any order, which means that the items stored in a set have no index, so you cannot access the elements by index, but you can access them by iteration.
- Mutable: Sets are mutable, which means that you can add or remove items from a set after it has been created.

Mappings
In Python, the mapping data type is used to store key-value pairs, where each key is associated with a value. The two main mapping data types in Python are:
- dict (dictionary): This is the most common and basic mapping data type in Python. It is used to store key-value pairs, where keys are unique and can be of any immutable type, such as a string or number. Dictionaries are defined using curly braces {} and items are separated by commas. Example: {‘a’: 1, ‘b’: 2, ‘c’: 3}, {1: ‘a’, 2: ‘b’, 3: ‘c’}
- defaultdict: This is a subclass of the built-in dict class. It is used when you want the keys in a dictionary to have a default value when they are first accessed. This is useful when you are trying to build a dictionary incrementally and don’t want to have to check if a key already exists in the dictionary. Example: defaultdict(int), defaultdict(list)
Boolean
This is a special data type that can take on one of two values: True or False.
None
This is a special data type that represents the absence of a value or a null value.
Binary
Bytes and bytearray are used to store binary data.
Others
There are also other less common data types in Python, such as arrays and memory views, which are used for more specialized tasks.
Conclusion
In conclusion, Python’s support for a variety of data types makes it a powerful tool for data manipulation and analysis. Understanding the different data types available in Python and how to use them is essential for any programmer looking to work with data in their programs. Whether you are working with simple data types like integers and strings or more complex data types like lists and dictionaries, Python’s support for different data types makes it a versatile language for data-driven applications. Java is another popular programming language and has similar data types to Python. It has its own java code compiler that you can use to run your code with ease. With the knowledge gained from this article, you can now confidently work with data in your Python programs and create powerful data-driven applications.